Woody ad snippets help you to execute PHP code, insert ad units or other external widget code anywhere in your WordPress website. Brief documentation gives an overview of all plugin features.
Installation
Brief description of different snippet types.
General Settings
Base Options
Activate by Default | The optional feature. If “ON” then all newly created snippets are active by default. |
Complete Uninstall | The optional feature. If “ON” then all data (snippets, settings) associated with the plugin will be removed after the plugin is uninstalled |
Code Editor
Code style | The optional feature. You can customize the code style in the snippet editor. The “Default” style is applied by default. |
Indent With Tabs | The optional feature. Whether, when indenting, the first N*tabSize spaces should be replaced by N tabs. The default is false. |
Tab Size | The optional feature. Pressing Tab in the code editor increases left indent to N spaces. N is a number pre-defined by you. |
Indent Unit | The optional feature. The indent for code lines (units). Example: select a snippet, press Tab. The left indent in the selected code increases to N spaces. N is a number pre-defined by you. |
Wrap Lines | The optional feature. If “ON” the editor will wrap long lines. Otherwise, it will create a horizontal scroll. |
Line Numbers | The optional feature. If “ON” all lines in the editor will be numbered. |
Auto Close Brackets | The optional feature. If “ON” the editor will automatically close opened quotes or brackets. Sometimes, it speeds up coding. |
Highlight Selection Matches | The optional feature. If “ON” it searches for matches for the selected variable/function name. Highlight matches with green. Improves readability. |
Creating Snippets
Snippets are short pieces of code or text that can be easily stored in WordPress admin bar and added to the page content. If you haven’t tried snippets yet, check out the benefits now:
- No code duplication (you no longer need to copy the code or text to several pages);
- Safety (no one except the admin can edit snippet’s content);
- Flexibility (you can place the code or text automatically using a special logic or entering shortcodes to the areas where the snippet is expected);
- Simple migration and website cleanup when you remove the plugin (you don’t need to go through all pages to remove code or a text snippet).
Let’s talk about snippet types, so you could choose the one you need.
Creating a PHP snippet
This is the easiest snippet type. It is used to execute PHP code only. If you want to execute any PHP code leaving your theme or the WordPress source code untouched then this is the type you need. Most users (perhaps, you are too!) still use function.php to place PHP code templates. That’s a bad idea with plenty of side effects. In fact, you get a messy function.php file and lose all changes with a regular theme update.
PHP snippets is a simple tool to modify, save and organize your PHP code in WordPress admin bar directly.
Setup
Let’s try to create your first PHP snippet.
Start with searching for a Woody snippets menu at the side menu.
Press +Add snippet.
You should see the following:
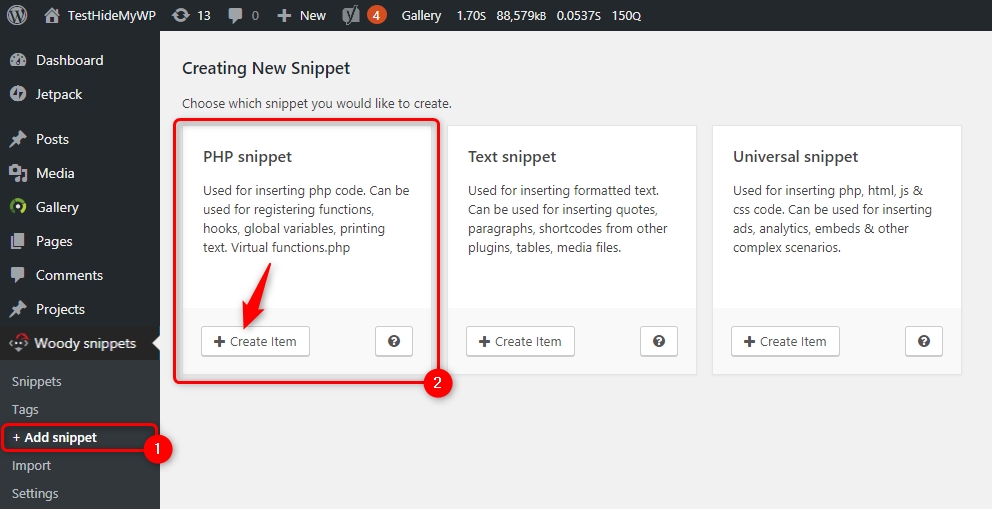
Select PHP Snippet as a snippet type and click Create item. The snippet settings will be opened.
Add a snippet title. Think of a logical name – this way it’ll be easier to find this snippet among others in the list.
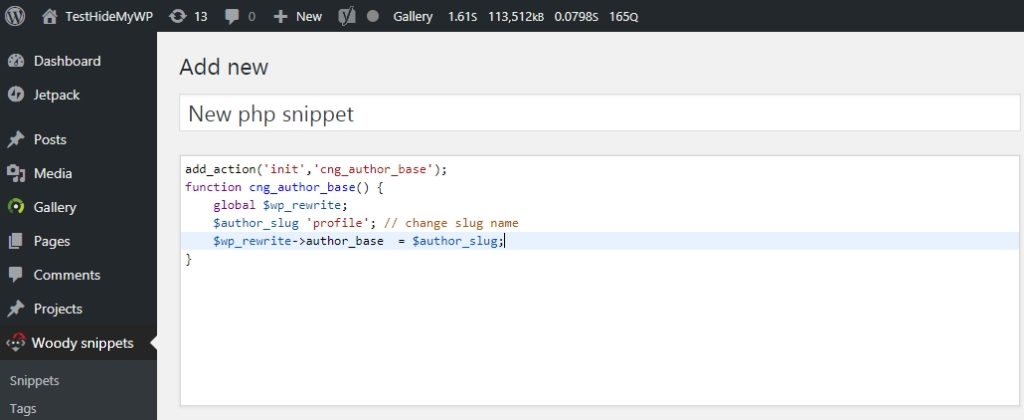
Most of the screen is occupied by the code editor with syntax highlighter. This is a multifunctional editor suitable for the HTML code as well. However, it’s better to use it for the PHP code.
Attention! The editor is used for a PHP code. This means you should add a PHP code without opening or closing PHP tags. You add a PHP code only. And the editor calculates what code to expect.

Use Cases for “everywhere”
Example #1: Registration of the ‘init‘ hook
add_action('init', 'cng_author_base'); function cng_author_base() { global $wp_rewrite; $author_slug = 'profile'; // change slug name $wp_rewrite->author_base = $author_slug; }
Use Cases for Shortcodes
Example #1: Show content to registered users only.
We’d like to hide a particular content from guests. This way we are motivating guests to sign up to our website. Let’s write a code that is going to solve this problem. The logic is pretty simple: if the is_user_logged_in” function exists & the user has signed in & any text has been sent to the $content variable, then we print the text from the $content variable (we’ll discuss how to add this text to a variable later).
if ( function_exists( 'is_user_logged_in' ) && is_user_logged_in() && ! empty( $content ) ) { echo $content; }
Now we need to wrap this special content in special shortcodes. Default attributes are: snippet id and snippet title.
[wbcr_php_snippet id="4034" title="Show content if a user has signed in"] Shortcodes can be used inside text widgets, in classic WordPress editor, Gutenberg editor, plugins and themes with shortcode support. [/wbcr_php_snippet]
Shortcodes can be used inside text widgets, in classic WordPress editor, Gutenberg editor, plugins and themes with shortcode support.
Attention! The content you wrap between shortcodes will always be available in the snippet via the $content variable. You should modify this variable. Otherwise, the content between shortcodes will be cut from the page content.
Example #2: Different Contact Form 7 layouts depend on the need_support parameter in the URL.
It’s a very popular question on our tech support forum: how to use another plugin shortcode in the PHP snippet? Let’s write a simple code that is going to display a contact form depends on the URL parameter. If a user follows the link https://site.loc/support?need_support=premium we’d like to show a contact form for the premium support. If a user clicks https://site.loc/support?need_support=freewe display a free support contact form. We create a condition: if the need_support request variable exists and it is not empty, we show the form type from the variable value. Otherwise, show the default form.
if(isset($_GET['need_support']) && !empty($_GET['need_support'])) { switch($_GET['need_support']) { case 'premium': echo do_shortcode('[contact-form-7 id="12" title="Need premium support"]'); break; case 'free': echo do_shortcode('[contact-form-7 id="11" title="Need free support"]'); break; case 'other': echo do_shortcode('[contact-form-7 id="10" title="Need other support"]'); break; default: echo do_shortcode('[contact-form-7 id="9" title="Default contact form"]'); break; } }
The do_shortcode feature helps to use shortcodes from other WordPress plugins.
Example #3: Calculate a number of days before the football game
We’ll show you a great example of how to avoid snippet duplication. We have a football website. Several games are expected soon. And we want to show how many days are left until each game begins. We only know dates of games. Some users would create individual snippets per each game. However, the only change here is the start date of the game. So no code duplication is necessary. We will need only one snippet. And we will add it using a shortcode. The shortcode’s start_date attribute sends the date of the game. Let’s write a simple code. It is going to calculate the number of days before the football game. This value is sent from the $start_date variable (see example below).
if ( isset( $start_date ) && ! empty( $start_date ) ) { $check_time = strtotime( $start_date ) - time(); if ( $check_time <= 0 ) { return false; } $days = floor( $check_time / 86400 ); if ( $days > 0 ) { echo $days . ' days'; } }
We print 3 games with countdowns of days. Each shortcode has a start_date attribute with the date of the game. And our PHP code handles the rest.
Days until Barcelona vs Madrid game: [wbcr_php_snippet id="4034" title="X days left till game #1" start_date="2019/01/25"] Days until Manchester United vs Bavaria game: [wbcr_php_snippet id="4034" title="X days left til game #2" start_date="2019/01/15"] Days until Chelsea vs Juventus game: [wbcr_php_snippet id="4034" title="X days left til game #3" start_date="2019/01/29"]
Creating a Text Snippet
The most simple snippet type. You can use text or HTML in there. Works like a classic TinyMCE editor. We’ve created this snippet type for simple text messages. You can use original post signatures, create frequently used quotes or cite documentation. This snippet type can insert a text unit on all pages automatically. Instead of making you do it manually for each new post.
Setup
Let’s create your first text snippet. Go to “Woody ad snippets” from the side menu and press “+Add snippet“. You should see the following :
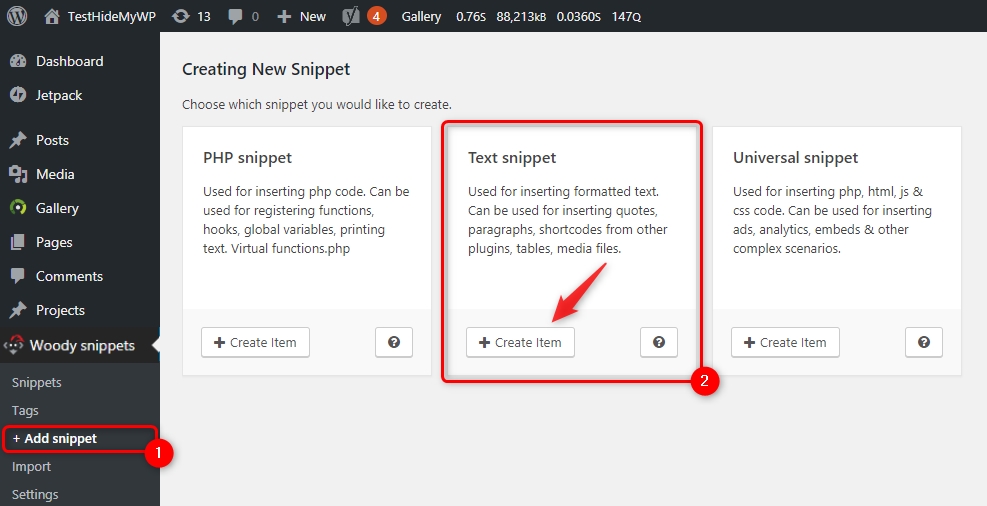
Add a snippet title. Think of a logical name – this way it’ll be easier to find this snippet among others in the list.
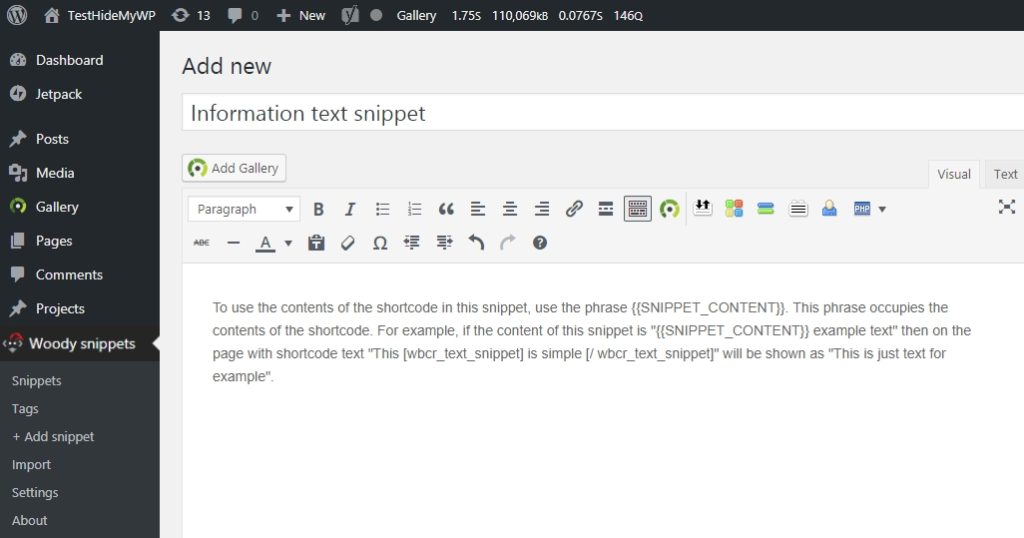
Creating a Universal Snippet
This is a complex snippet type, where you can combine PHP, HTML, JavaScript, and CSS. We’ve designed this snippet type as a handy way of inserting ad codes, widgets from external services, complex HTML forms, Google analytics, Yandex metrics, Facebook pixels and so on. You can place universal snippets on all pages automatically based on the conditional logic.
Setup
Let’s create your first universal snippet. Go to “Woody ad snippets “ from the side menu and press “+Add snippet“. You should see the following:
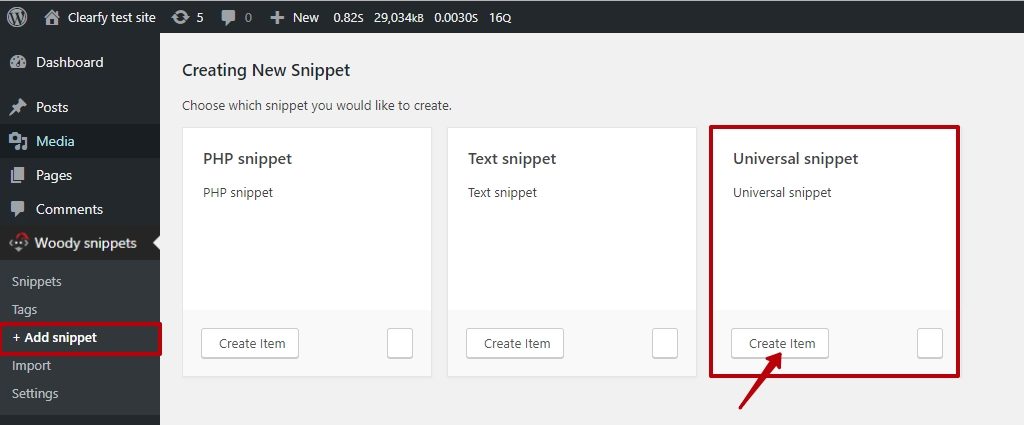
Add a snippet title. Think of a logical name – this way it’ll be easier to find this snippet among others in the list.
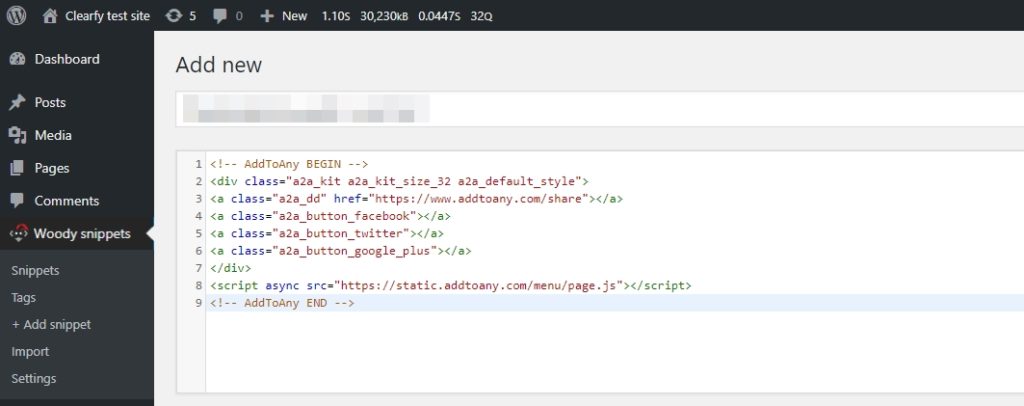
Additional Settings
Description – you can add a short description of a snippet. This way your colleagues or administrators can understand what this particular snippet or text does.
Add a snippet title. Think of a logical name – this way it’ll be easier to find this snippet among others in the list.
Available attributes – you can define a list of attributes sent to the snippet through shortcodes. Use a comma as a separator. Example: we will add two attributes place and user, to the field. Now shortcodes of this snippet can get two new attributes [wbcr_php_snippet id=”4034″ place=”home_page” user=”admin”]. Their values are passed as $place and $user variables.
Placement options
Everywhere (for php snippets) – registration of functions, classes, hooks, and global variables. For example, when you plan to use a PHP function in other snippets, but don’t want to write code for each snippet. In this case, you just create a new snippet and choose the placement option “Everywhere”. Now your function is available on all website. This option is similar to function.php. If you place the code to the function.php file, you’ll get the same effect.
Shortcode – can print any content on a certain page or inside a widget. The applicability is wide. For example, if you want to show how many days are left before the football game or hide some content from unregistered users.
Automatic insertion (for universal and text snippets only) – Automatically inserts text and universal snippets anywhere on your website. It’s quite handy if you want to insert an add code after each post or page.
Placement Scopes
All website:
- Header – snippet is added to the source code before the tag. You can also make a sticky header in just a few clicks
- Footer – snippet is added to the source code before the tag.
Posts, pages, custom posts:
- Before Post – snippet is added to the source code before the tag.
- Before Content – snippet is added before the post/page content.
- Before Paragraph – snippet is added before a certain paragraph. A paragraph number is listed in the Location number field.
- After Paragraph – snippet is added after a certain paragraph. A paragraph number is listed in the Location number field.
- After Content – snippet is added after the post/page content.
- After Post – snippet is added after the post/page.
Categories, archives, tags, taxonomy:
- Before Excerpt – snippet is added before the post/page preview.
- After Excerpt – snippet is added after the post/page preview.
- Between Posts – snippet is added between posts.
- Before post – snippet is added before a certain post. A post number is listed in the Location number field.
- After post – snippet is added after a certain post. A post number is listed in the Location number field.
Display options
Brief description of snippet types.
How to Add a Snippet Shortcode in a Text Widget?
You can use snippet shortcodes in custom text widgets. It’s useful whenever you insert any ad unit to the sidebar or footer.
- Go to “Widgets”
- Add a new text widget to the sidebar;
- If you need to move widget content to a snippet select the content inside the snippet. Otherwise, just set the cursor in the text field.
- Click the plugin icon at the widget toolbar and select any snippet. Only active snippets with Shortcodes placement options are available at the list.
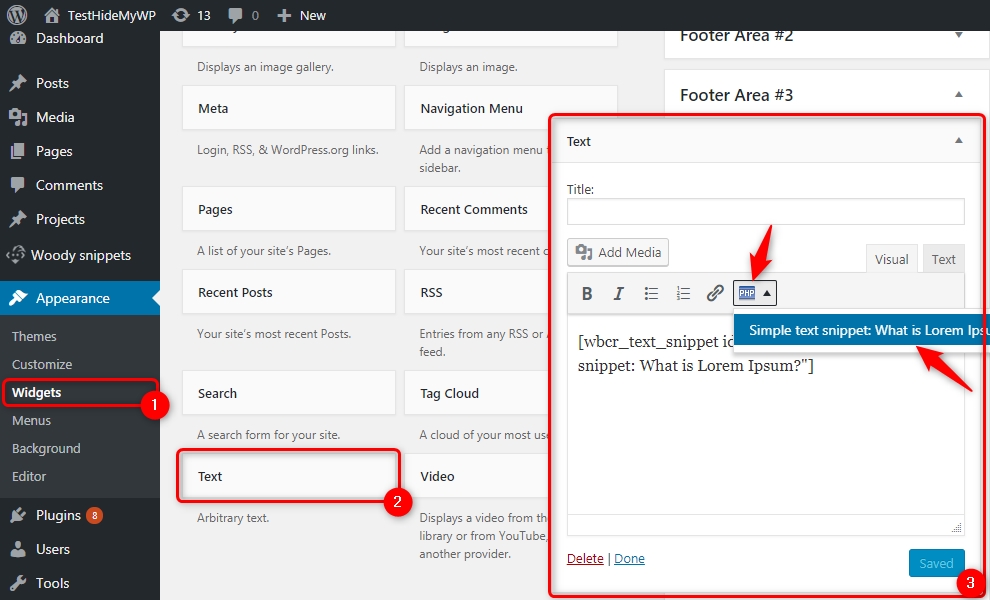
How to Add a Snippet Shortcode in a Classic Editor?
You can use snippet shortcodes inside posts, pages and custom posts. Here’s an example of inserting a snippet shortcode to a classic WordPress editor.
- Go to “All posts” section;
- Select a post to modify;
- If you need to move widget content to a snippet select the content inside the snippet. Otherwise, just set the cursor in the text field.
- Click the plugin icon at the widget toolbar and select any snippet. Only active snippets with Shortcodes placement options are available at the list.
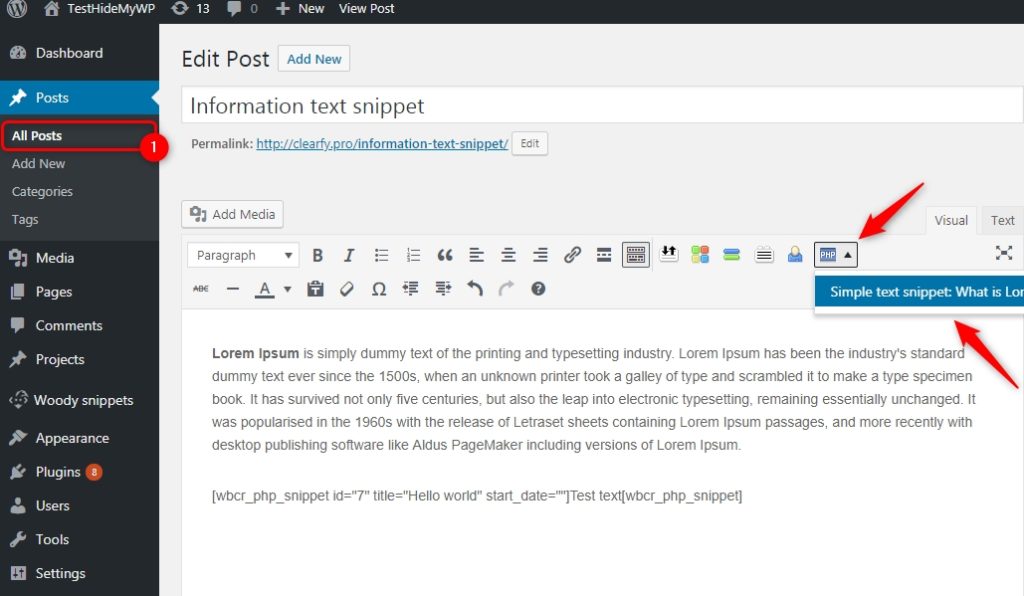
How to Add a Snippet Shortcode in the Gutenberg Editor?
Creating the content becomes more simple and easy. You can forget about shortcodes to locate snippet and switch to the user-friendly blocks instead. Just create a snippet with the location scope through the shortcode, go to Gutenberg Editor and add a new Woody ad snippet block.
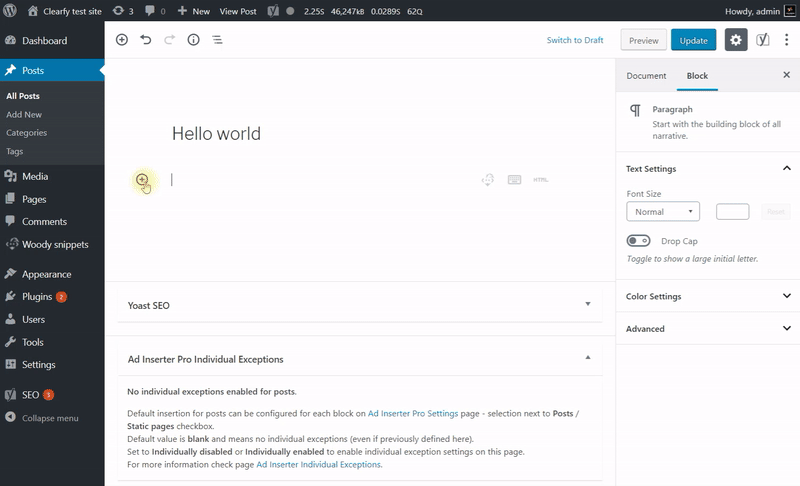
How to Move a PHP Code from the Old Plugin Version (1.3) to a New One (2.x.x)?
The old version of Insert php 1.3.0 worked this way: you wrapped PHP code with shortcodes [insert_php]php code[/insert_php]. Starting from version 2.x.x and higher, you need to create special snippets to place PHP code. Use a snippet shortcode [wbcr_php_snippetid=”xx”] instead of shortcodes [insert_php][/insert_php] to execute PHP code on pages.
We recommend you to move all your code from the post editor to snippets.
Important! TinyMCE converts double quotes to special characters. So if you place this code on the snippet editor, it may not work. To avoid this problem, replace all special symbols of double quotes in your PHP code with classic double quotes.
You have a multilingual website built with WPML and want to host code snippets with Woody Ad Snippets. How to do it?
- Create a new code snippet in Woody or open an already created snippet. It can be any snippet, Universal snippet, php snippet, etc.
- This is the snippet code, scroll down
- You need a WPML Language block
- Check the languages you want and save the snippet
- Done. Now the snippet will be displayed only on the required language version of your site. Woody Ad Snippets has conditional logic for displaying snippets, don’t worry, it doesn’t conflict with the language selection.